CODESYS V3 supports all IEC-61131-3 operators. These operators are recognized implicitly throughout the project. In addition to these IEC operators, CODESYS also supports some non-IEC 61131-3 operators.
Operators are used in blocks, such as functions.
For information about the processing order (binding strength) of the ST operators, please refer to the section on ST expressions.
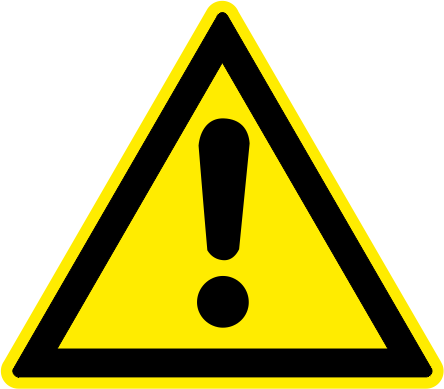
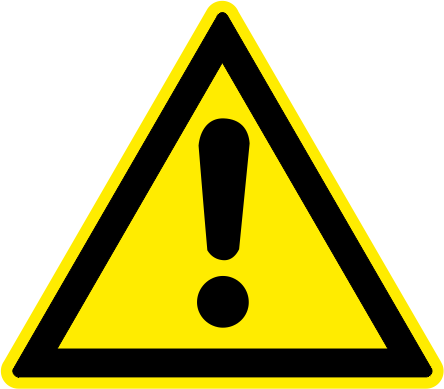
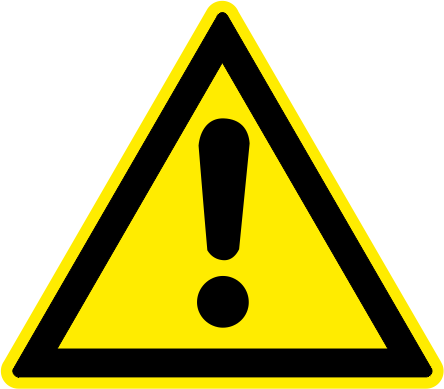
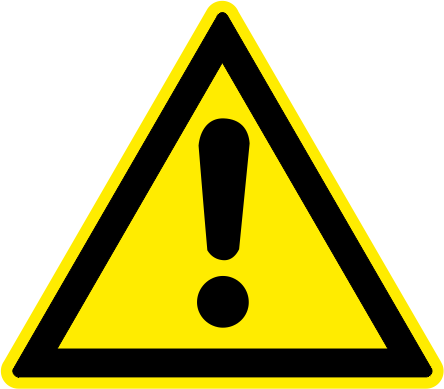
CAUTION
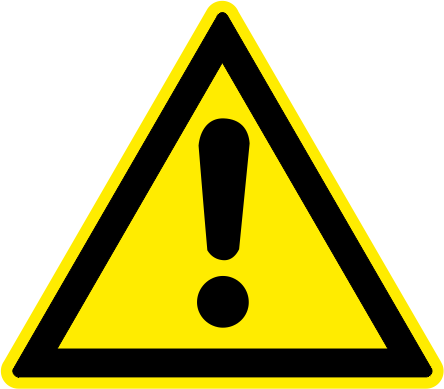
For operations with floating-point data types, the computational result depends on the applied target system hardware.
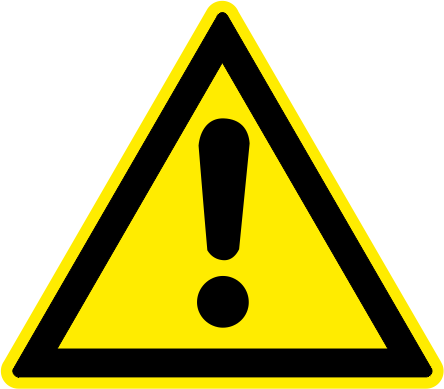
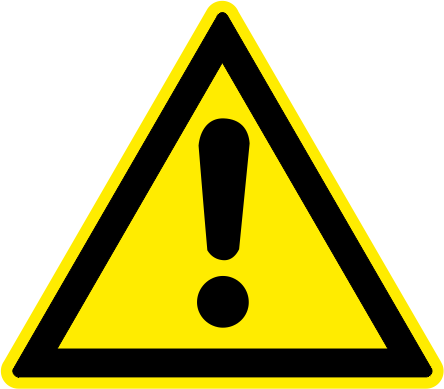
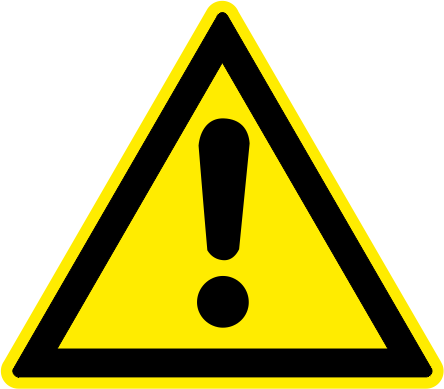
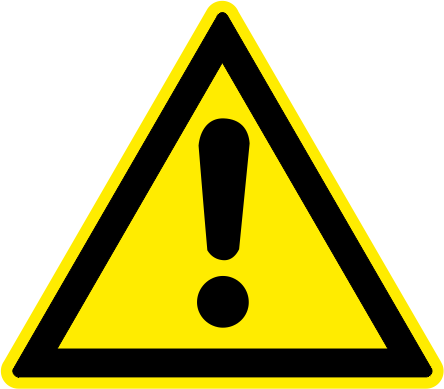
CAUTION
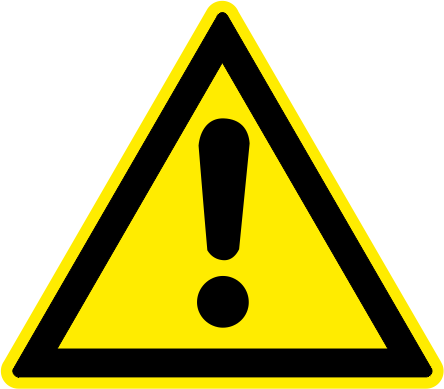
For operations with overflow or underflow in the data type, the computational result depends on the applied target system hardware.
Overflow/underflow in the data type
The CODESYS compiler generates code for the target device and computes temporary results always with the native size that is defined by the target device. For example, computation is performed at least with 32-bit temporary values on x86 and ARM systems and always with 64-bit temporary values on x64 systems. This provides considerable advantages in the computation speed and often also produces the desired result. But this also means that an overflow or underflow in the data type is not truncated in some cases.
Examples
Example 1
The result of this addition is not truncated and the result in dwVar
is 65536
.
VAR wVar : WORD; dwVar: DWORD; END_VAR wVar := 65535; dwVar := wVar + 1;
Example 2
The overflow and underflow in the data type is not truncated and the results (bVar1, bVar2
) of both comparisons are FALSE
on 32-bit and 64-bit hardware.
VAR wVar1 : WORD; wVar2 : WORD; bVar1 : BOOL; bVar2 : BOOL; END_VAR wVar1 := 65535; wVar2 := 0; bVar1 := (wVar1 + 1) = wVar2; bVar2 := (wVar2 - 1) = wVar1;
Example 3
By the assignment to wVar3
, the value is truncated to the target data type WORD
and the result bvar1
is TRUE
.
VAR wVar1 : WORD; wVar2 : WORD; wVar3 : WORD; bVar1 : BOOL; END_VAR wVar1 := 65535; wVar2 := 0; wVar3 := (wVar1 + 1); bVar1 := wVar3 = wVar2;
Example 4
In order to force the compiler to truncate the temporary results, a conversion can be inserted.
The type conversion makes sure that both comparisons are 16-bit only and the results
(bVar1, bVar2
) of both comparisons are each TRUE
.
VAR wVar1 : WORD; wVar2 : WORD; bVar1 : BOOL; bVar2 : BOOL; END_VAR wVar1 := 65535; wVar2 := 0; bVar1 := TO_WORD(wVar1 + 1) = wVar2; bVar2 := TO_WORD(wVar2 - 1) = wVar1;
Arithmetic operators
Bitstring operators
Selection operators
Comparison operators
A comparison operator is a Boolean that compares two inputs (first and second operand).
Call operators
Type conversion operators
You can explicitly call type conversion operators. The type conversion operators described
below are available for typed conversions from one elementary type to another elementary
type, as well as for overloading. Conversions from a larger type to a smaller type
are also implicitly possible (for example, from INT
to BYTE
or from DINT
to WORD
).
Typed conversion: <elementary data type> _TO_ <another elementary data type>
Overloaded conversion: TO_ <elementary data type>
Elementary data types:
<elementary data type> = __UXINT | __XINT | __XWORD | BIT | BOOL | BYTE | DATE | DATE_AND_TIME | DINT | DT | DWORD | INT | LDATE | LDATE_AND_TIME | LDT | LINT | LREAL | LTIME | LTOD | LWORD | REAL | SINT | TIME | TOD | UDINT | UINT | ULINT | USINT | WORD
The keywords T
, TIME_OF_DAY
and DATE_AND_TIME
are alternative forms for the data types TIME
, TOD
, and DT
. T
, TIME_OF_DAY
and DATE_AND_TIME
are not represented as a type conversion command.
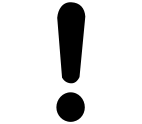
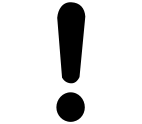
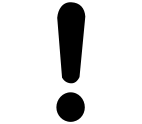
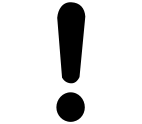
NOTICE
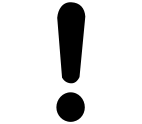
If the operand value for a type conversion operator is outside of the value range
of the target data type, then the result output depends on the processor type and
is therefore undefined. This is the case, for example, when a negative operand value
is converted from LREAL
to the target data type UINT
.
Information can be lost when converting from larger data types to smaller data types.
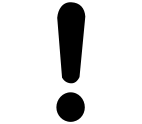
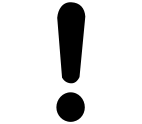
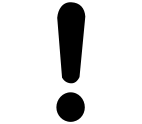
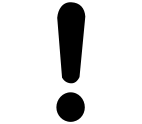
NOTICE
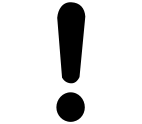
String manipulation when converting to STRING
or WSTRING
When converting the type to STRING
or WSTRING
, the typed value is left-aligned as a character string and truncated if it is too
long. Therefore, declare the return variable for the type conversion operators <>_TO_STRING
and <>_TO_WSTRING
long enough that the character string has enough space without any manipulation.
Numeric Operators
Namespace operators
Namespace operators are extended from IEC 61131-3 operators. They make it possible for you to provided unique access to variables and modules, even when you use the same name multiple times for variables or modules in a project.
⮫ “Operator - Global namespace”
⮫ “Operator - Namespace for global variables lists”
⮫ “Operator - Enumeration namespace”
Multicore operators
Working with different tasks requires the synchronization of these tasks. This is especially true when working on multicore platforms. Some special operators are provided in CODESYS to support this synchronization.
These operators are extensions of IEC-61131-3. The operators TEST_AND_SET
and __COMPARE_AND_SWAP
are used for similar tasks.