A pointer stores the memory address of objects, such as variables or function block instances, at runtime.
Syntax of the pointer declaration:
<pointer name>: POINTER TO <data type> | <data unit type> | <function block name>
;
Example
FUNCTION_BLOCK FB_Point VAR piNumber: POINTER TO INT; iNumber1: INT := 5; iNumber2: INT; END_VAR piNumber := ADR(iNumber1); // piNumber is assigned to address of iNumber1 iNumber2 := piNumber^; // value 5 of iNumber1 is assigned to variable iNumber2 by dereferencing of pointer piNumber
Dereferencing a pointer means obtaining the value to which the pointer points. A pointer
is dereferenced by appending the content operator ^
to the pointer identifier (example:, piNumber^
in the example above). To assign the address of an object to a pointer, the address
operator ADR
is applied to the object: ADR(iNumber1)
.
In online mode, you can use ⮫ “Command: Go to Reference ” to jump from a pointer to the declaration location of the referenced variable.
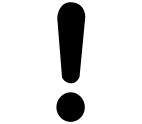
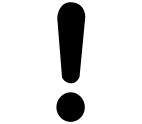
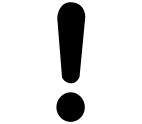
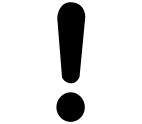
NOTICE
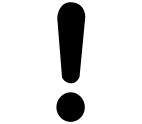
When a pointer points to an I/O input, write access applies. When the code is generated, this leads to the compiler
warning “'<pointer name>' is not a valid assignment target”. Example: pwInput := ADR(wInput);
If you require a construct of this kind, you have to first copy the input value (wInput
) to a variable with write access.
-
Index access to pointers
-
Subtracting pointers