The purpose of conditional pragmas is to influence the generation of code in the pre-compilation process or the compilation process. The ST implementation language supports these pragmas.
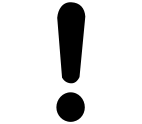
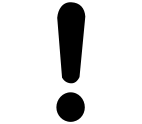
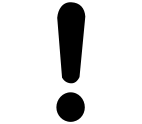
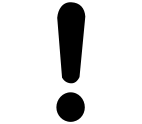
NOTICE
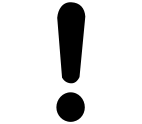
They use conditional pragmas in the implementations of POUs. CODESYS does not evaluate these conditional pragmas if you use them in the declaration part.
With conditional pragmas you affect whether implementation code is taken into account for the compilation. For example, you can make this dependent on whether a certain variable is declared, whether a certain function block exists, etc.
Pragma |
Description |
---|---|
|
The value can be queried and compared later with |
|
The |
|
These are pragmas for the conditional compilation. The specified expressions |
|
You can use one or more operators within the constant expression |
You can enter expressions and define
definitions as “compiler definitions” in the “Build” tab in the Properties dialog of POUs. If you enter define
definitions in the properties dialog, you must omit the term {define}
, contrary to the definition in the implementation code. In addition, you can specify
several define
definitions in the properties dialog, separated by commas.
Operator defined (<identifier>)
This operator causes the expression to be given the value TRUE
. The requirement is that the identifier <identifier>
was defined with the help of a {define}
instruction and not undefined again afterwards with an {undefine}
instruction; otherwise FALSE
is returned.
Example
Requirement: The applications App1
and App2
exist. The variable pdef1
is defined by a {define}
statement in App1
, but not in App2
.
{IF defined (pdef1)} (* This code is processed in App1 *) {info 'pdef1 defined'} hugo := hugo + SINT#1; {ELSE} (* the following code is only processed in App2 *) {info 'pdef1 not defined'} hugo := hugo - SINT#1; {END_IF}
This also contains an example of a message pragma: Only the message pdef1 defined
is displayed in the message view when the application is compiled, because pdef1
is actually defined. The message pdef1 not defined
is displayed if pdef1
is not defined.
Operator defined (variable: <variable_name>)
This operator causes the expression to be given the value TRUE
if the variable <variable_name>
is declared within the current scope; otherwise FALSE
is returned.
Example
Requirement: The two applications App1
and App2
exist. The variable g_bTest
is declared in App1
, but not in App2
.
{IF defined (variable: g_bTest)} (* the following code is only processed in App2*) g_bTest := x > 300; {END_IF}
Operator defined (type: <identifier>)
The operator causes the expression to be given the value TRUE
if a data type is declared with the identifier <identifier>
; otherwise FALSE
is returned.
Example
Requirement: The two applications App1
and App2
exist. The data type DUT
is declared in App1
, but not in App2
.
{IF defined (type: DUT)} (* the following code is only processed in App1*) bDutDefined := TRUE; {END_IF}
Operator defined (pou: <pou name>)
The operator causes the expression to be given the value TRUE
if a function block or an action with name <pou-name>
exists; otherwise FALSE
is returned.
Example
Requirement: The two applications App1
and App2
exist. The function block CheckBounds
exists in App1
, but not in App2
.
{IF defined (pou: CheckBounds)} (* the following code is only processed in App1 *) arrTest[CheckBounds(0,i,10)] := arrTest[CheckBounds(0,i,10)] + 1; {ELSE} (* the following code is only processed in App2 *) arrTest[i] := arrTest[i]+1; {END_IF}
Operator defined (task: <task_name>)
Not yet implemented!
The operator causes the expression to be given the value TRUE
if a task is defined with the name <task_name>
; otherwise FALSE
is returned.
Syntax
{ IF | ELSIF defined (task: <task name> }
Example
{IF defined (task: Task_D)}
Example
Requirement: The two applications App1
and App2
exist. The task PLC_PRG_Task
is defined in App1
, but not in App2
.
{END_IF} {IF defined (task: PLC_PRG_Task)} (* the following code is only processed in App1 *) erg := plc_prg.x; {ELSE} (* the following code is only processed in App2 *) erg := prog.x; {END_IF}
Operator defined (resource: <identifier>)
Not yet implemented!
The operator causes the expression to be given the value TRUE
if a resource object with the name <identifier>
exists for the application; otherwise FALSE
is returned.
Example
Requirement: The two applications App1
and App2
exist. A resource object glob_var1
of the global variable list exists for App1
, but not for App2
.
{IF defined (resource:glob_var1)} (* the following code is only processed in App1 *) gvar_x := gvar_x + ivar; {ELSE} (* the following code is only processed in App2 *) x := x + ivar; {END_IF}
Operator defined (IsSimulationMode)
The operator causes the expression to be given the value TRUE
if the application runs on a simulated device, i.e. in simulation mode.
See also
Operator defined (IsLittleEndian)
The operator causes the expression to be given the value FALSE
, if the CPU memory is organized in Big Endian (Motorola byte order).
Operator defined (IsFPUSupported)
If the expression returns the value TRUE
, then the code generator produces an FPU code (for the floating-point unit processor)
when calculating with REAL
values. Otherwise CODESYS emulates FPU operations, which is much slower.
Operator hasattribute (pou: <pou name>, '<attribute>')
This operator causes the expression to be given the value TRUE
if the attribute <attribute>
is specified in the first line of the declaration part of the function block <pou name>
; otherwise FALSE
is returned.
Example
Requirement: The two applications App1
and App2
exist. The function fun1
is declared in App1
and App2
. However, in App1
it is also provided with the pragma {attribute 'vision'}
.
In App1
:
{attribute 'vision'} FUNCTION fun1 : INT VAR_INPUT i : INT; END_VAR VAR END_VAR
In App2
:
FUNCTION fun1 : INT VAR_INPUT i : INT; END_VAR VAR END_VAR
Pragma instruction:
{IF hasattribute (pou: fun1, 'vision')} (* the following code is only processed in App1 *) ergvar := fun1(ivar); {END_IF}
See also
Operator hasattribute (variable: <variable>, '<attribute>')
This operator causes the expression to be given the value TRUE
if the pragma {attribute '<attribute>'}
is assigned to the variable in the line before the variable declaration; otherwise
FALSE
is returned.
Example
Requirement: The two applications App1
and App2
exist. The variable g_globalInt
is used in App1
and App2
, but in App1
the attribute 'DoCount'
is assigned to it in addition.
Declaration of g_GlobalInt
in App1
VAR_GLOBAL {attribute 'DoCount'} g_globalInt : INT; g_multiType : STRING; END_VAR
Declaration g_GlobalInt
in App2
:
VAR_GLOBAL g_globalInt : INT; g_multiType : STRING; END_VAR
Pragma instruction:
{IF hasattribute (variable: g_globalInt, 'DoCount')} (* the following code is only processed in App1 *) g_globalInt := g_globalInt + 1; {END_IF}
See also
Operator hasconstanttype (constant name, boolean literal)
The operator checks whether or not the constant, which is identified with <constant name>, has been replaced. The second parameter (Boolean value) controls what is checked:
-
TRUE: Checks if the constant has been replaced
-
FALSE: Checks if the constant has not been replaced When the respective case occurs, the operator returns TRUE.
Syntax
{ IF hasconstanttype( <constant namne> , <boolean literal> ) } { ELSIF hasconstanttype( <constant namne> , <boolean literal> ) }
Example
{IF hasconstanttype(PLC_PRG.aConst, TRUE)}
The automatic replacement of constants in principle depends on the following:
-
Compile option
-
Replace constants
-
Constant type (For example, STRING types are never replaced.)
-
Usage of the attribute {attribute 'const_non_replaced'}
-
Usage of the attribute {attribute 'const_replaced'}
Example
VAR iCntMAXIsReplaced: INT; xErrorOccured : BOOL; END_VAR VAR CONSTANT c_iMAX: INT := 99; END_VAR {IF hasconstanttype(c_iMAX, TRUE)} iCntMAXIsReplaced := iCntMAXIsReplaced + 1; {ELSE} xErrorOccured := FALSE; {END_IF}
Operator hasconstantvalue (constant name, variable name, comparison operator)
The operator compares the value of the constant, which is identified with <constant name>, with the value of the second parameter. The second parameter can be specified either as a literal <literal> or as a variable <variable name>.
Comparison operators <comparison operator>:
-
Greater than (>)
-
Greater than or equal to (>=)
-
Equal to (=)
-
Not equal to (<>)
-
Less than or equal to (<=)
-
Less than (<)
Syntax
{ IF hasconstantvalue( <constant name> , <variable name> , <comparison operator> ) { IF hasconstantvalue( <constant name> , <literal> , <comparison operator> ) { ELSIF hasconstantvalue( <constant name> , <variable name> , <comparison operator> ) { ELSIF hasconstantvalue( <constant name> , <literal> , <comparison operator> )
Example
{IF hasconstantvalue(PLC_PRG.aConst, 99, >)} {ELSIF hasconstantvalue(PLC_PRG.aConst, GVL.intconst99, =)}
Example
PROGRAM PRG_ConditionConstantValue VAR iCntMAX: INT; iCntGlobalMAX : INT; iCntABC: INT; iCntGlobalABC : INT; xErrorOccured : BOOL; END_VAR VAR CONSTANT c_iMAX: INT := 999; c_sABC: STRING := 'ABC'; {attribute 'const_non_replaced'} c_iNonReplaceable: INT := 888; END_VAR {IF hasconstantvalue(c_iMAX, 999, =)} iCntMAX := iCntMAX + 1; {ELSE} xErrorOccured := FALSE; {END_IF} {IF hasconstantvalue(c_iMAX, GVL.gc_iMAX, =)} iCntGlobalMAX := iCntGlobalMAX + 1; {ELSE} xErrorOccured := FALSE; {END_IF} {IF hasconstantvalue(c_sABC, 'ABC', =)} iCntABC := iCntMAX + 1; {ELSE} xErrorOccured := FALSE; {END_IF} {IF hasconstantvalue(c_sABC, GVL.gc_sABC, =)} iCntGlobalABC := iCntMAX + 1; {ELSE} xErrorOccured := FALSE; {END_IF}
Operator hastype (variable: <variable>, <type-spec>)
This operator causes the expression to be given the value TRUE
if the variable <variable>
is of the data type <type-spec>
; otherwise FALSE
is returned.
Possible data types for <type-spec>
:
-
BOOL
-
BYTE
-
DATE
-
DATE_AND_TIME (DT)
-
DINT
-
DWORD
-
INT
-
LDATE
-
LDATE_AND_TIME (LDT)
-
LINT
-
LREAL
-
LTIME
-
LTIME_OF_DAY (LTOD)
-
LWORD
-
REAL
-
SINT
-
STRING
-
TIME
-
TIME_OF_DAY (TOD)
-
ULINT
-
UDINT
-
UINT
-
USINT
-
WORD
-
WSTRING
Example
Requirement: The two applications App1
and App2
exist. The variable g_multitype
is declared in App1
with data type LREAL
, in App2
with data type STRING
.
{IF (hastype (variable: g_multitype, LREAL))} (* the following code is only processed in App1 *) g_multitype := (0.9 + g_multitype) * 1.1; {ELSIF (hastype (variable: g_multitype, STRING))} (* the following code is only processed in App2 *) g_multitype := 'this is a multitalent'; {END_IF}
Operator hasvalue (PackMode, '<pack mode value>')
The checked pack mode depends on the device description, not on the pragma that can be specified for individual DUTs.
Operator hasvalue (RegisterSize, '<register size>')
<register size>: Size of a CPU register (in bits) The operator causes the expression to return the value TRUE when the size of a CPU register is equal to <register size>.
Possible values for <register size>
-
16 for C16x
-
64 for X86-64 bit
-
32 for X86-32 bit
Operator hasvalue (<define-ident>, '<char-string>')
This operator causes the expression to be given the value TRUE
if a variable is defined with the identifier <define-ident>
and has the value <char-string>
; otherwise FALSE
is returned.
Example
Requirement: The two applications App1
and App2
exist. The variable test
is used in the applications App1
and App2
; in App1
it is given the value 1
, in App2
the value 2
.
{IF hasvalue(test,'1')} (* the following code is only processed in App1 *) x := x + 1; {ELSIF hasvalue(test,'2')} (* the following code is only processed in App2 *) x := x + 2; {END_IF}
Operator NOT <operator>
The expression is given the value TRUE
if the reverse value of <operator>
returns the value TRUE
. <operator>
can be one of the operators described in this chapter.
Example
Requirement: The two applications App1
and App2
exist.PLC_PRG1
exists in App1
and App2
, and the POU CheckBounds
exists only in App1
.
{IF defined (pou: PLC_PRG1) AND NOT (defined (pou: CheckBounds))} (* the following code is only processed in App2 *) bANDNotTest := TRUE; {END_IF}
Operator <operator> AND <operator>
The expression is given the value TRUE
if the two specified operators return TRUE
. <operator>
can be one of the operators described in this chapter.
Example
Requirement: The applications App1
and App2
exist.PLC_PRG1
exists in App1
and App2
, the POU CheckBounds
only in App1
.
{IF defined (pou: PLC_PRG1) AND (defined (pou: CheckBounds))} (* the following code is only processed in App1 *) bANDTest := TRUE; {END_IF}
Operator <operator> OR <operator>
The expression returns TRUE
if one of the two specified operators returns TRUE
. <operator>
can be one of the operators described in this chapter.
Example
Requirement: The two applications App1
and App2
exist. The POU PLC_PRG1
exists in App1
and App2
, and the POU CheckBounds
exists only in App1
.
{IF defined (pou: PLC_PRG1) OR (defined (pou: CheckBounds))} (* the following code is only processed in App1 and in App2 *) bORTest := TRUE; {END_IF}
Operator (<operator>)
()
parenthesizes the operators.
See also