A reference implicitly refers to another object. When accessed, the reference is implicitly
dereferenced, and therefore does not need a special content operator ^
such as a pointer.
Syntax
<identifier> : REFERENCE TO <data type> ; <data type>: base type of the reference
Example
PROGRAM PLC_PRG VAR rspeA : REFERENCE TO DUT_SPECIAL; pspeA : POINTER TO DUT_SPECIAL; speB : DUT_SPECIAL; END_VAR rspeA REF= speB; // Reference rspeA is alias for speB. The code corresponds to pspeA := ADR(speB); rspeA := speD; // The code corresponds to pspeA^ := speB;
The readability of a program is made difficult when the same memory cell is accessed
simultaneously by means of an identifier and its alias (for example, speB
and rspeA
).
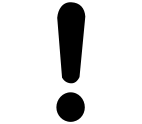
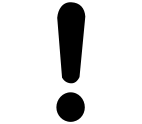
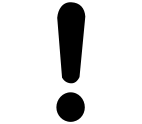
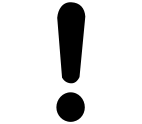
NOTICE
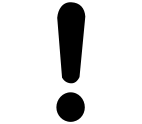
With compiler version >= V3.3.0.0, references are initialized (at 0).
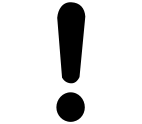
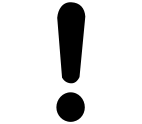
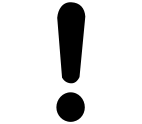
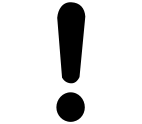
NOTICE
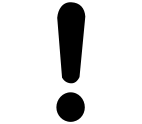
If a reference refers to a device input, then the access (for example, rInput REF= Input;
) is applies as write access. This leads to a compiler warning when the code is generated:
"...invalid assignment target
".
If you require a construct of this kind, you have to first copy the input value (rInput
) to a variable with write access.
Invalid declarations
ariTest : ARRAY[0..9] OF REFERENCE TO INT; priTest : POINTER TO REFERENCE TO INT; rriTest : REFERENCE TO REFERENCE TO INT; rbitTest : REFERENCE TO BIT;
A reference type must not be used as the base type of an array, pointer, or reference. Furthermore, a reference must not refer to a bit variable. These kinds of constructs generate compiler errors.
Comparison of reference and pointer
A reference has the following advantages over a pointer:
-
Easier to use:
A reference can access the contents of the referenced object directly and without dereferencing.
-
Finer and simpler syntax when passing values:
Call of a function block which passes a reference without an address operator instead of a pointer
Example:
fbDoIt(riInput:=iValue);
Instead of:
fbDoIt_1(piInput:=ADR(iValue));
-
Type safety:
When assigning two references, the compiler checks whether their base types match. This is not checked in the case of pointers.
Testing the validity of a reference
You can use the operator __ISVALIDREF
to check whether or not a reference points to a valid value (meaning a value not
equal to 0).
Syntax
<Boolean variable name> := __ISVALIDREF( <reference name> );
<reference name>
: Identifier declared with REFERENCE TO
The Boolean variable is TRUE
when the reference points to a valid value. Otherwise it is FALSE
.
Example
PROGRAM PLC_PRG VAR iAlfa : INT; riBravo : REFERENCE TO INT; riCharlie : REFERENCE TO INT; bIsRef_Bravo : BOOL := FALSE; bIsRef_Charlie : BOOL := FALSE; END_VAR iAlfa := iAlfa + 1; riBravo REF= iAlfa; riCharlie REF= 0; bIsRef_Bravo := __ISVALIDREF(riBravo); (* becomes TRUE, because riBravo references to iAlfa, which is non-zero *) bIsRef_Charlie := __ISVALIDREF(riCharlie); (* becomes FALSE, because riCharlie is set to 0 *)
In compiler version 3.5.7.40 and later, the implicit monitoring function “CheckPointer” acts on variables of type REFERENCE TO
in the same way as for pointer variables.