An array is a collection of data elements of the same data type. CODESYS supports one- and multi-dimensional arrays of fixed or variable length.
As of Automation Builder 2.6.0 array initializations within type definitions are no longer allowed.
Array of fixed length
You can define arrays in the declaration part of a POU or in global variable lists.
Syntax of the declaration of a one-dimensional array
<variable name> : ARRAY[ <dimension> ] OF <data type> ( := <initialization> )? ; <dimension> : <lower index bound>..<upper index bound> <data type> : elementary data types | user defined data types | function block types // (...)? : Optional
Syntax of the declaration of a multi-dimensional array
<variable name> : ARRAY[ <1st dimension> ( , <next dimension> )+ ] OF <data type> ( := <initialization> )? ; <1st dimension> : <1st lower index bound>..<1st upper index bound> <next dimension> : <next lower index bound>..<next upper index bound> <data type> : elementary data types | user defined data types | function block types // (...)+ : One or more further dimensions // (...)? : Optional
The index limits are integers; maximum of the data type DINT
.
Syntax for data access
<variable name>[ <index of 1st dimension> ( , <index of next dimension> )* ] // (...)* : 0, one or more further dimensions
Note the capability of using the implicit monitoring function CheckBounds()
to monitor the maintenance of the index limits at runtime.
Example
One-dimensional array of 10 integer elements
VAR aiCounter : ARRAY[0..9] OF INT; END_VAR
Lower index limit: 0
Upper index limit: 9
Initialization
aiCounter : ARRAY[0..9] OF INT := [0, 10, 20, 30, 40, 50, 60, 70, 80, 90];
Data access
iLocalVariable := aiCounter[2];
The value 20 is assigned to the local variable.
Example
2-dimensional array
VAR aiCardGame : ARRAY[1..2, 3..4] OF INT; END_VAR
1st dimension: 1 to 2
2nd dimension: 3 to 4
Initialization
aiCardGame : ARRAY[1..2, 3..4] OF INT := [2(10),2(20)]; // Short notation for [10, 10, 20, 20]
Data access
iLocal_1 := aiCardGame[1, 3]; // Assignment of 10 iLocal_2 := aiCardGame[2, 4]; // Assignment of 20
Example
3-dimensional array
VAR aiCardGame : ARRAY[1..2, 3..4, 5..6] OF INT; END_VAR
1st dimension: 1 to 2
2nd dimension: 3 to 4
3rd dimension: 5 to 6
2 * 2 * 2 = 8 array elements
Initialization
aiCardGame : ARRAY[1..2, 3..4, 5..6] OF INT := [10, 20, 30, 40, 50, 60, 70, 80];
Data access
iLocal_1 := aiCardGame[1, 3, 5]; // Assignment of 10 iLocal_2 := aiCardGame[2, 3, 5]; // Assignment of 20 iLocal_3 := aiCardGame[1, 4, 5]; // Assignment of 30 iLocal_4 := aiCardGame[2, 4, 5]; // Assignment of 40 iLocal_5 := aiCardGame[1, 3, 6]; // Assignment of 50 iLocal_6 := aiCardGame[2, 3, 6]; // Assignment of 60 iLocal_7 := aiCardGame[1, 4, 6]; // Assignment of 70 iLocal_8 := aiCardGame[2, 4, 6]; // Assignment of 80
Initialization
aiCardGame : ARRAY[1..2, 3..4, 5..6] OF INT := [2(10), 2(20), 2(30), 2(40)]; // Short notation for [10, 10, 20, 20, 30, 30, 40, 40]
Data access
iLocal_1 := aiCardGame[1, 3, 5]; // Assignment of 10 iLocal_2 := aiCardGame[2, 3, 5]; // Assignment of 10 iLocal_3 := aiCardGame[1, 4, 5]; // Assignment of 20 iLocal_4 := aiCardGame[2, 4, 5]; // Assignment of 20 iLocal_5 := aiCardGame[1, 3, 6]; // Assignment of 30 iLocal_6 := aiCardGame[2, 3, 6]; // Assignment of 30 iLocal_7 := aiCardGame[1, 4, 6]; // Assignment of 40 iLocal_8 := aiCardGame[2, 4, 6]; // Assignment of 40
Example
3-dimensional arrays of a user-defined structure
TYPE DATA_A STRUCT iA_1 : INT; iA_2 : INT; dwA_3 : DWORD; END_STRUCT END_TYPE PROGRAM PLC_PRG VAR aData_A : ARRAY[1..3, 1..3, 1..10] OF DATA_A; END_VAR
The array aData_A
consists of a total of 3 * 3 * 10 = 90 array elements of data type DATA_A
.
Initialize partially
aData_A : ARRAY[1..3, 1..3, 1..10] OF DATA_A := [(iA_1 := 1, iA_2 := 10, dwA_3 := 16#00FF),(iA_1 := 2, iA_2 := 20, dwA_3 := 16#FF00),(iA_1 := 3, iA_2 := 30, dwA_3 := 16#FFFF)];
In the example, only the first 3 elements are initialized explicitly. Elements to
which no initialization value is assigned explicitly are initialized internally with
the default value of the basic data type. This initializes the structure components
at 0 starting with the element aData_A[2, 1, 1]
.
Data access
iLocal_1 := aData_A[1,1,1].iA_1; // Assignment of 1 dwLocal_2 := aData_A[3,1,1].dwA_3; // Assignment of 16#FFFF
Example
Array of a function block
FUNCTION BLOCK FBObject_A VAR iCounter : INT; END_VAR ... PROGRAM PLC_PRG VAR aObject_A : ARRAY[1..4] OF FBObject_A; END_VAR
The array aObject_A
consists of 4 elements. Each element instantiates a FBObject_A
function block.
Function call
aObject_A[2]();
Example
Implementation of FB_Something
with method FB_Init
FUNCTION_BLOCK FB_Something VAR _nId : INT; _lrIn : LREAL; END_VAR ... METHOD FB_Init : BOOL VAR_INPUT bInitRetains : BOOL; bInCopyCode : BOOL; nId : INT; lrIn : LREAL; END_VAR _nId := nId; _lrIn := lrIN;
The function block FB_Something
has a method FB_Init
that requires 2 parameters.
Instantiation of the array with initialization
PROGRAM PLC_PRG VAR fb_Something_1 : FB_Something(nId := 11, lrIn := 33.44); a_Something : ARRAY[0..1, 0..1] OF FB_Something[(nId := 12, lrIn := 11.22), (nId := 13, lrIn := 22.33), (nId := 14, lrIn := 33.55),(nId := 15, lrIn := 11.22)]; END_VAR
Array of arrays
The declaration of an "array of arrays" is an alternative syntax for multidimensional arrays. A collection of elements is nested instead of dimensioning the elements. The nesting depth is unlimited.
Syntax for declaration
<variable name> : ARRAY[<first>] ( OF ARRAY[<next>] )+ OF <data type> ( := <initialization> )? ; <first> : <first lower index bound>..<first upper index bound> <next> : <lower index bound>..<upper index bound> // one or more arrays <data type> : elementary data types | user defined data types | function block types // (...)+ : One or more further arrays // (...)? : Optional
Syntax for data access
<variable name>[<index of first array>] ( [<index of next array>] )+ ; // (...)* : 0, one or more further arrays
Example
PROGRAM PLC_PRG VAR aiPoints : ARRAY[1..2,1..3] OF INT := [1,2,3,4,5,6]; ai2Boxes : ARRAY[1..2] OF ARRAY[1..3] OF INT := [ [1, 2, 3], [ 4, 5, 6]]; ai3Boxes : ARRAY[1..2] OF ARRAY[1..3] OF ARRAY[1..4] OF INT := [ [ [1, 2, 3, 4], [5, 6, 7, 8 ], [9, 10, 11, 12] ], [ [13, 14, 15, 16], [ 17, 18, 19, 20], [21, 22, 23, 24] ] ]; ai4Boxes : ARRAY[1..2] OF ARRAY[1..3] OF ARRAY[1..4] OF ARRAY[1..5] OF INT; END_VAR aiPoints[1, 2] := 1200; ai2Boxes[1][2] := 1200;
The variables aiPoints
and ai2Boxes
collect the same data elements, however the syntax for the declaration differs from
that of the data access.
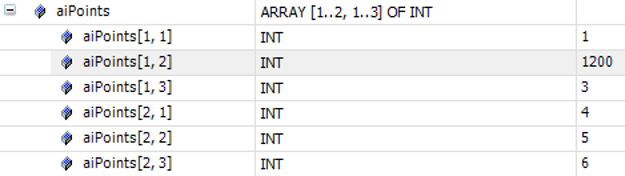
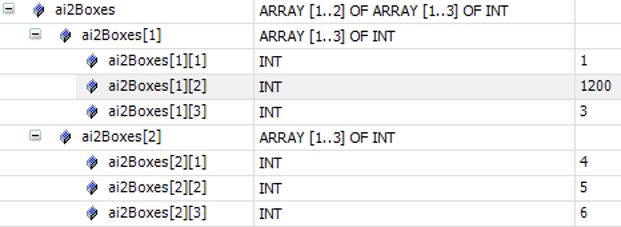
Array of variable length
In function blocks, functions, or methods, you can declare arrays of variable length
in the VAR_IN_OUT
declaration section.
The LOWER_BOUND
and UPPER_BOUND
operators are provided for determining the index limits of the actual used array
at runtime.
Only statically declared arrays (not arrays generated by means of the operator __NEW
) may be passed to an array with variable length.
Syntax of the declaration of a one-dimensional array of variable length
<variable name> : ARRAY[*] OF <data type> ( := <initialization> )? ; <data type> : elementary data types | user defined data types | function block types // (...)? : Optional
Syntax of the declaration of a multi-dimensional array of variable length
<variable name> : ARRAY[* ( , * )+ ] OF <data type> ( := <initialization> )? ; <data type> : elementary data types | user defined data types | function block types // (...)+ : One or more further dimensions // (...)? : Optional
Syntax of the operators for calculating the limit index
LOWER_BOUND( <variable name> , <dimension number> ) UPPER_BOUND( <variable name> , <dimension number> )
Example
The SUM
function adds the integer values of the array elements and returns the calculated
sum as a result. The sum is calculated across all array elements available at runtime.
As the actual number of array elements will only be known at runtime, the local variable
is declared as a one-dimensional array of variable length.
FUNCTION SUM: INT; VAR_IN_OUT aiData : ARRAY[*] OF INT; END_VAR VAR diCounter : DINT; iResult : INT; END_VAR iResult := 0; FOR diCounter := LOWER_BOUND(aiData, 1) TO UPPER_BOUND(aiData, 1) DO // Calculates the length of the current array iResult := iResult + aiData[diCounter]; END_FOR; SUM := iResult;